qm-dsp
1.8
|
TempoTrackV2.h
Go to the documentation of this file.
void viterbi_decode(const d_mat_t &rcfmat, const d_vec_t &wv, d_vec_t &bp, d_vec_t &tempi)
Definition: TempoTrackV2.cpp:231
!! Question: how far is this actually sample rate dependent? I
Definition: TempoTrackV2.h:26
void adapt_thresh(d_vec_t &df)
void calculateBeatPeriod(const std::vector< double > &df, std::vector< double > &beatPeriod, std::vector< double > &tempi)
Definition: TempoTrackV2.h:41
double mean_array(const d_vec_t &dfin, int start, int end)
void get_rcf(const d_vec_t &dfframe, const d_vec_t &wv, d_vec_t &rcf)
Definition: TempoTrackV2.cpp:176
TempoTrackV2(float sampleRate, int dfIncrement)
Construct a tempo tracker that will operate on beat detection function data calculated from audio at ...
Definition: TempoTrackV2.cpp:28
void calculateBeats(const std::vector< double > &df, const std::vector< double > &beatPeriod, std::vector< double > &beats)
Definition: TempoTrackV2.h:56
Generated by
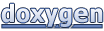