qm-dsp
1.8
|
TCSgram.h
Go to the documentation of this file.
Definition: TCSgram.h:27
void normalize()
Definition: TonalEstimator.h:53
void setFrameDuration(const double dFrameDurationMS)
Definition: TCSgram.h:40
Generated by
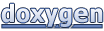