qm-dsp
1.8
|
MedianFilter.h
Go to the documentation of this file.
50 std::cerr << "WARNING: MedianFilter::push: attempt to push NaN, pushing zero instead" << std::endl;
MedianFilter & operator=(const MedianFilter &)
MedianFilter(int size, float percentile=50.f)
Definition: MedianFilter.h:28
static std::vector< T > filter(int size, const std::vector< T > &in)
Definition: MedianFilter.h:81
Definition: MedianFilter.h:25
Generated by
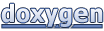