qm-dsp
1.8
|
KLDivergence.cpp
Go to the documentation of this file.
double distanceDistribution(const std::vector< double > &d1, const std::vector< double > &d2, bool symmetrised)
Calculate a Kullback-Leibler divergence of two probability distributions.
Definition: KLDivergence.cpp:47
double distanceGaussian(const std::vector< double > &means1, const std::vector< double > &variances1, const std::vector< double > &means2, const std::vector< double > &variances2)
Calculate a symmetrised Kullback-Leibler divergence of Gaussian models based on mean and variance vec...
Definition: KLDivergence.cpp:22
Generated by
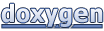