qm-dsp
1.8
|
BeatSpectrum.cpp
Go to the documentation of this file.
double distance(const std::vector< double > &v1, const std::vector< double > &v2)
Definition: CosineDistance.cpp:24
Definition: CosineDistance.h:21
std::vector< double > process(const std::vector< std::vector< double > > &inmatrix)
Definition: BeatSpectrum.cpp:22
Generated by
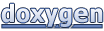