FFmpeg
|
VP8 compatible video decoder. More...
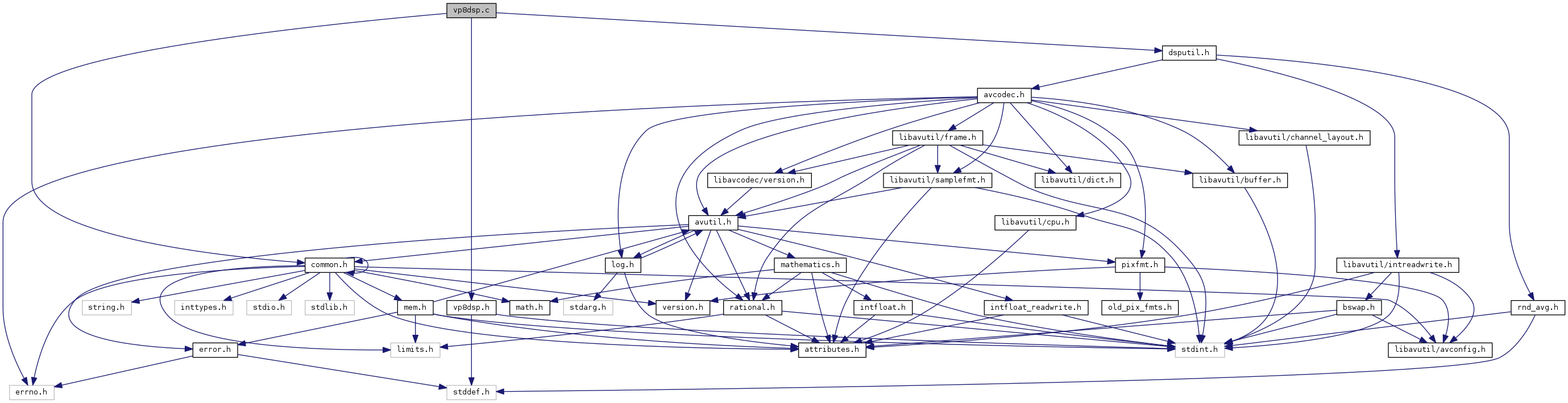
Go to the source code of this file.
Macros | |
#define | MUL_20091(a) ((((a)*20091) >> 16) + (a)) |
#define | MUL_35468(a) (((a)*35468) >> 16) |
#define | LOAD_PIXELS |
#define | clip_int8(n) (cm[n+0x80]-0x80) |
#define | LOOP_FILTER(dir, size, stridea, strideb, maybe_inline) |
#define | UV_LOOP_FILTER(dir, stridea, strideb) |
#define | PUT_PIXELS(WIDTH) |
#define | FILTER_6TAP(src, F, stride) |
#define | FILTER_4TAP(src, F, stride) |
#define | VP8_EPEL_H(SIZE, TAPS) |
#define | VP8_EPEL_V(SIZE, TAPS) |
#define | VP8_EPEL_HV(SIZE, HTAPS, VTAPS) |
#define | VP8_BILINEAR(SIZE) |
#define | VP8_MC_FUNC(IDX, SIZE) |
#define | VP8_BILINEAR_MC_FUNC(IDX, SIZE) |
Functions | |
static void | vp8_luma_dc_wht_c (int16_t block[4][4][16], int16_t dc[16]) |
static void | vp8_luma_dc_wht_dc_c (int16_t block[4][4][16], int16_t dc[16]) |
static void | vp8_idct_add_c (uint8_t *dst, int16_t block[16], ptrdiff_t stride) |
static void | vp8_idct_dc_add_c (uint8_t *dst, int16_t block[16], ptrdiff_t stride) |
static void | vp8_idct_dc_add4uv_c (uint8_t *dst, int16_t block[4][16], ptrdiff_t stride) |
static void | vp8_idct_dc_add4y_c (uint8_t *dst, int16_t block[4][16], ptrdiff_t stride) |
static av_always_inline void | filter_common (uint8_t *p, ptrdiff_t stride, int is4tap) |
static av_always_inline int | simple_limit (uint8_t *p, ptrdiff_t stride, int flim) |
static av_always_inline int | normal_limit (uint8_t *p, ptrdiff_t stride, int E, int I) |
E - limit at the macroblock edge I - limit for interior difference. More... | |
static av_always_inline int | hev (uint8_t *p, ptrdiff_t stride, int thresh) |
static av_always_inline void | filter_mbedge (uint8_t *p, ptrdiff_t stride) |
static void | vp8_v_loop_filter_simple_c (uint8_t *dst, ptrdiff_t stride, int flim) |
static void | vp8_h_loop_filter_simple_c (uint8_t *dst, ptrdiff_t stride, int flim) |
av_cold void | ff_vp8dsp_init (VP8DSPContext *dsp) |
Variables | |
static const uint8_t | subpel_filters [7][6] |
Detailed Description
VP8 compatible video decoder.
Definition in file vp8dsp.c.
Macro Definition Documentation
Definition at line 157 of file vp8dsp.c.
Referenced by filter_common(), and filter_mbedge().
#define LOAD_PIXELS |
Definition at line 147 of file vp8dsp.c.
Referenced by filter_common(), filter_mbedge(), hev(), normal_limit(), and simple_limit().
#define LOOP_FILTER | ( | dir, | |
size, | |||
stridea, | |||
strideb, | |||
maybe_inline | |||
) |
Definition at line 78 of file vp8dsp.c.
Referenced by vp8_idct_add_c().
Definition at line 79 of file vp8dsp.c.
Referenced by vp8_idct_add_c().
#define PUT_PIXELS | ( | WIDTH | ) |
#define UV_LOOP_FILTER | ( | dir, | |
stridea, | |||
strideb | |||
) |
#define VP8_BILINEAR_MC_FUNC | ( | IDX, | |
SIZE | |||
) |
Definition at line 483 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
#define VP8_EPEL_H | ( | SIZE, | |
TAPS | |||
) |
#define VP8_EPEL_HV | ( | SIZE, | |
HTAPS, | |||
VTAPS | |||
) |
#define VP8_EPEL_V | ( | SIZE, | |
TAPS | |||
) |
#define VP8_MC_FUNC | ( | IDX, | |
SIZE | |||
) |
Definition at line 472 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Function Documentation
av_cold void ff_vp8dsp_init | ( | VP8DSPContext * | dsp | ) |
Definition at line 494 of file vp8dsp.c.
Referenced by vp8_decode_init().
|
static |
Definition at line 159 of file vp8dsp.c.
Referenced by vp8_h_loop_filter_simple_c(), and vp8_v_loop_filter_simple_c().
|
static |
|
static |
|
static |
|
static |
Definition at line 190 of file vp8dsp.c.
Referenced by normal_limit(), vp8_h_loop_filter_simple_c(), and vp8_v_loop_filter_simple_c().
Definition at line 297 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Definition at line 81 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Definition at line 130 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Definition at line 138 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Definition at line 116 of file vp8dsp.c.
Referenced by ff_vp8dsp_init(), vp8_idct_dc_add4uv_c(), and vp8_idct_dc_add4y_c().
|
static |
Definition at line 32 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
|
static |
Definition at line 65 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Definition at line 288 of file vp8dsp.c.
Referenced by ff_vp8dsp_init().
Variable Documentation
Generated on Thu Jun 5 2025 06:56:57 for FFmpeg by
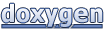