FFmpeg
|
gsmdec_template.c
Go to the documentation of this file.
static void short_term_synth(GSMContext *ctx, int16_t *dst, const int16_t *src)
Definition: gsmdec_template.c:81
About Git write you should know how to use GIT properly Luckily Git comes with excellent documentation git help man git shows you the available git< command > help man git< command > shows information about the subcommand< command > The most comprehensive manual is the website Git Reference visit they are quite exhaustive You do not need a special username or password All you need is to provide a ssh public key to the Git server admin What follows now is a basic introduction to Git and some FFmpeg specific guidelines Read it at least if you are granted commit privileges to the FFmpeg project you are expected to be familiar with these rules I if not You can get git from etc no matter how small Every one of them has been saved from looking like a fool by this many times It s very easy for stray debug output or cosmetic modifications to slip in
Definition: git-howto.txt:5
static int decode_log_area(int coded, int factor, int offset)
Definition: gsmdec_template.c:54
bitstream reader API header.
static void apcm_dequant_add(GetBitContext *gb, int16_t *dst)
Definition: gsmdec_template.c:31
static void long_term_synth(int16_t *dst, int lag, int gain_idx)
Definition: gsmdec_template.c:45
static int gsm_decode_block(AVCodecContext *avctx, int16_t *samples, GetBitContext *gb)
Definition: gsmdec_template.c:120
Definition: gsmdec_data.h:28
Definition: get_bits.h:54
Filter the word “frame” indicates either a video frame or a group of audio samples
Definition: filter_design.txt:2
Generated on Wed Apr 16 2025 06:53:15 for FFmpeg by
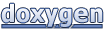