Constant-Q Library
|
#include <CQSpectrogram.h>
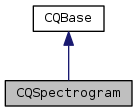
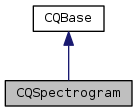
Public Types | |
enum | Interpolation { InterpolateZeros, InterpolateHold, InterpolateLinear } |
![]() | |
typedef std::complex< double > | Complex |
A single complex-valued sample. More... | |
typedef std::vector< double > | RealSequence |
A series of real-valued samples ordered in time. More... | |
typedef std::vector< double > | RealColumn |
A series of real-valued samples ordered by bin (frequency or similar). More... | |
typedef std::vector< Complex > | ComplexSequence |
A series of complex-valued samples ordered in time. More... | |
typedef std::vector< Complex > | ComplexColumn |
A series of complex-valued samples ordered by bin (frequency or similar). More... | |
typedef std::vector< RealColumn > | RealBlock |
A matrix of real-valued samples, indexed by time then bin number. More... | |
typedef std::vector< ComplexColumn > | ComplexBlock |
A matrix of complex-valued samples, indexed by time then bin number. More... | |
Public Member Functions | |
CQSpectrogram (CQParameters params, Interpolation interpolation) | |
virtual | ~CQSpectrogram () |
virtual bool | isValid () const |
virtual double | getSampleRate () const |
virtual int | getBinsPerOctave () const |
virtual int | getOctaves () const |
virtual int | getTotalBins () const |
virtual int | getColumnHop () const |
virtual int | getLatency () const |
virtual double | getMaxFrequency () const |
virtual double | getMinFrequency () const |
virtual double | getBinFrequency (double bin) const |
RealBlock | process (const RealSequence &) |
RealBlock | getRemainingOutput () |
Detailed Description
Calculate a dense constant-Q magnitude spectrogram from time-domain input. The input of each process call is a single frame of time-domain samples; the output is a series of fixed-height columns. See process for details.
If you need the full complex-valued constant-Q output, you must use the ConstantQ class instead.
Member Enumeration Documentation
Constructor & Destructor Documentation
CQSpectrogram::CQSpectrogram | ( | CQParameters | params, |
Interpolation | interpolation | ||
) |
Construct a Constant-Q magnitude spectrogram object using the given transform parameters.
|
virtual |
Member Function Documentation
|
inlinevirtual |
Return the frequency of a given bin in the Constant-Q output. This actually maps a continuous "bin scale" value to frequency: the bin parameter does not have to be an integer.
Implements CQBase.
|
inlinevirtual |
Return the number of bins per octave specified when constructing the Constant-Q implementation.
Implements CQBase.
|
inlinevirtual |
Return the spacing, in samples at the sample rate returned from getSampleRate(), between one column and the next.
Implements CQBase.
|
inlinevirtual |
Return the latency of Constant-Q calculation, in samples at the sample rate returned from getSampleRate().
Implements CQBase.
|
inlinevirtual |
Return the maximum frequency of the Constant-Q output, i.e. the frequency of the highest bin in the output. This will normally be the same as the maximum frequency passed to the constructor of the specific Constant-Q implementation.
Implements CQBase.
|
inlinevirtual |
Return the minimum frequency of the Constant-Q output, i.e. the frequency of the lowest bin in the output. This is derived from the maximum frequency and octave count, and is not necessarily the same as any minimum frequency requested when constructing the Constant-Q implementation.
Implements CQBase.
|
inlinevirtual |
Return the number of octaves spanned by the Constant-Q transform.
Implements CQBase.
CQSpectrogram::RealBlock CQSpectrogram::getRemainingOutput | ( | ) |
Return the remaining constant-Q magnitude columns following the end of processing. Any buffered input is padded so as to ensure that all input provided to process() will have been returned.
|
inlinevirtual |
Return the sample rate used when constructing the specific Constant-Q implementation.
Implements CQBase.
|
inlinevirtual |
Return the total number of bins in each Constant-Q column (i.e. bins-per-octave times number of octaves).
Implements CQBase.
|
inlinevirtual |
Return true if the Constant-Q implementation was successfully constructed, with a valid set of initialisation parameters.
Implements CQBase.
CQSpectrogram::RealBlock CQSpectrogram::process | ( | const RealSequence & | td | ) |
Given a series of time-domain samples, return a series of constant-Q magnitude columns. Any samples left over (that did not fit into a constant-Q processing block) are saved for the next call to process or getRemainingBlocks.
The input is assumed to be a single frame of time-domain sample values, such that consecutive calls to process receive contiguous frames from the source signal. Each frame may be of any length in samples.
Each output column contains a series of constant-Q bin value magnitudes, ordered from highest to lowest frequency.
The columns are all of the same height, but they might not all be populated, depending on the interpolation mode: in InterpolateZeros mode, the lower octaves (which are spaced more widely in the raw constant-Q than the highest octave) will contain zeros for the undefined values, but in the other interpolation modes every cell will be filled.
To obtain raw, complex constant-Q bin values, use the ConstantQ class.
The documentation for this class was generated from the following files:
Generated on Thu May 16 2019 06:33:03 for Constant-Q Library by
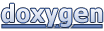