Constant-Q Library
|
#include <CQBase.h>
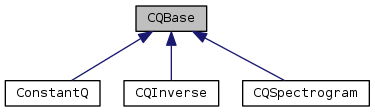
Public Types | |
typedef std::complex< double > | Complex |
A single complex-valued sample. More... | |
typedef std::vector< double > | RealSequence |
A series of real-valued samples ordered in time. More... | |
typedef std::vector< double > | RealColumn |
A series of real-valued samples ordered by bin (frequency or similar). More... | |
typedef std::vector< Complex > | ComplexSequence |
A series of complex-valued samples ordered in time. More... | |
typedef std::vector< Complex > | ComplexColumn |
A series of complex-valued samples ordered by bin (frequency or similar). More... | |
typedef std::vector< RealColumn > | RealBlock |
A matrix of real-valued samples, indexed by time then bin number. More... | |
typedef std::vector< ComplexColumn > | ComplexBlock |
A matrix of complex-valued samples, indexed by time then bin number. More... | |
Public Member Functions | |
virtual bool | isValid () const =0 |
virtual double | getSampleRate () const =0 |
virtual int | getBinsPerOctave () const =0 |
virtual int | getOctaves () const =0 |
virtual int | getTotalBins () const =0 |
virtual int | getColumnHop () const =0 |
virtual int | getLatency () const =0 |
virtual double | getMaxFrequency () const =0 |
virtual double | getMinFrequency () const =0 |
virtual double | getBinFrequency (double bin) const =0 |
Detailed Description
Interface class for Constant-Q implementations, containing common type declarations and means to query configuration parameters.
Member Typedef Documentation
typedef std::complex<double> CQBase::Complex |
A single complex-valued sample.
typedef std::vector<ComplexColumn> CQBase::ComplexBlock |
A matrix of complex-valued samples, indexed by time then bin number.
typedef std::vector<Complex> CQBase::ComplexColumn |
A series of complex-valued samples ordered by bin (frequency or similar).
typedef std::vector<Complex> CQBase::ComplexSequence |
A series of complex-valued samples ordered in time.
typedef std::vector<RealColumn> CQBase::RealBlock |
A matrix of real-valued samples, indexed by time then bin number.
typedef std::vector<double> CQBase::RealColumn |
A series of real-valued samples ordered by bin (frequency or similar).
typedef std::vector<double> CQBase::RealSequence |
A series of real-valued samples ordered in time.
Member Function Documentation
|
pure virtual |
Return the frequency of a given bin in the Constant-Q output. This actually maps a continuous "bin scale" value to frequency: the bin parameter does not have to be an integer.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the number of bins per octave specified when constructing the Constant-Q implementation.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the spacing, in samples at the sample rate returned from getSampleRate(), between one column and the next.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the latency of Constant-Q calculation, in samples at the sample rate returned from getSampleRate().
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the maximum frequency of the Constant-Q output, i.e. the frequency of the highest bin in the output. This will normally be the same as the maximum frequency passed to the constructor of the specific Constant-Q implementation.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the minimum frequency of the Constant-Q output, i.e. the frequency of the lowest bin in the output. This is derived from the maximum frequency and octave count, and is not necessarily the same as any minimum frequency requested when constructing the Constant-Q implementation.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the number of octaves spanned by the Constant-Q transform.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the sample rate used when constructing the specific Constant-Q implementation.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return the total number of bins in each Constant-Q column (i.e. bins-per-octave times number of octaves).
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
|
pure virtual |
Return true if the Constant-Q implementation was successfully constructed, with a valid set of initialisation parameters.
Implemented in CQInverse, CQSpectrogram, and ConstantQ.
The documentation for this class was generated from the following file:
Generated on Thu May 16 2019 06:33:03 for Constant-Q Library by
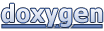