Bela
|
#include <DigitalChannelManager.h>
Public Member Functions | |
void | setCallback (void(*newCallback)(bool, unsigned int, void *)) |
void | setCallbackArgument (unsigned int channel, void *arg) |
void | processInput (uint32_t *array, unsigned int length) |
void | processOutput (uint32_t *array, unsigned int length) |
bool | isSignalRate (unsigned int channel) |
bool | isMessageRate (unsigned int channel) |
bool | isInput (unsigned int channel) |
bool | isOutput (unsigned int channel) |
void | setValue (unsigned int channel, bool value) |
void | unmanage (unsigned int channel) |
void | manage (unsigned int channel, bool direction, bool isMessageRate) |
Detailed Description
This class manages the digital channels.
Each channel can be set as either signal or message rate. When set as message rate: inputs are parsed when calling processInput() and a callback is invoked when they change state, outputs are set via calls to setValue() and then written to the output array when calling processOutput().
When set at signal rate: the DigitalChannelManager only sets the pin direction.
Throughout the class we keep track of message/signal rate and input/output in separate variables, so that we always know if a channel is managed by the DigitalChannelManager or not. This way, managed and unmanaged channels can be freely mixed in the code.
For the reasons above, isSignalRate(channel) is not the same as !isMessageRate(channel) and isInput(channel) is not the same as !isOutput(channel)
Member Function Documentation
|
inline |
Set the callback.
The callback will be called when one of the managed message-rate input bits changes state. The callback's signature is void (stateChangedCallback)(bool value, unsigned int delay, void arg) where value
is the new value of the channel, delay
is the sample in the most recent block when the status changed, and arg
is an additional argument passed to the callback, which can be set for each channel using setCallbackArgument()
|
inline |
Sets the argument for the callback.
Typically an argument will allow the callback to identify the channel that changed state.
- Parameters
-
channel is the channel for which the argument is set arg is the argument that will be passed to the callback for that channel
|
inline |
Process the input signals.
Parses the input array and looks for state changes in the bits managed as message-rate inputs and invokes the appropriate callbacks.
- Parameters
-
array the array of input values length the length of the array
|
inline |
Process the output signals.
Processes the output array and appropriately sets the bits managed as message-rate outputs. Bits marked as input and unmanaged bits are left alone.
It also updates the channel directions.
- Parameters
-
array the array of input values length the length of the array
|
inline |
Inquires if the channel is managed as signal-rate.
- Parameters
-
channel the channel which is inquired.
|
inline |
Inquires if the channel is managed as message-rate.
- Parameters
-
channel the channel which is inquired.
- Returns
- true if the channel is managed at message-rate, false otherwise
|
inline |
Inquires if the channel is managed as input.
- Parameters
-
channel the channel which is inquired.
- Returns
- true if the channel is managed as input, false otherwise
|
inline |
Inquires if the channel is managed as output.
- Parameters
-
channel the channel which is inquired.
- Returns
- true if the channel is managed as output, false otherwise
|
inline |
Sets the output value for a channel.
- Parameters
-
channel the channel to set. value the value to set.
|
inline |
Stops managing a channel.
- Parameters
-
channel the channel number
|
inline |
Manages a channel.
Starts managing a channel with given direction and rate. It can be called repeatedly on a given channel to change direction and/or rate.
- Parameters
-
channel the channel to manage. direction
The documentation for this class was generated from the following file:
Generated on Mon Mar 10 2025 06:27:56 for Bela by
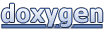