Bela
|
PulseIn.h
60 int hasPulsed(BelaContext* context, int frame){//let's leave this in PulseIn.h to allow the compiler to optimize out the call.
61 if(_lastContext != context->audioFramesElapsed){ // check for pulses in the whole context and cache the result
Structure holding current audio and sensor settings and pointers to data buffers. ...
Definition: Bela.h:190
void init(BelaContext *context, unsigned int digitalInput, int direction=1)
Definition: PulseIn.h:13
void check(BelaContext *context)
const uint64_t audioFramesElapsed
Number of elapsed audio frames since the start of rendering.
Definition: Bela.h:271
Main Bela public API.
Generated on Sat May 31 2025 06:27:57 for Bela by
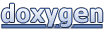