Bela
|
PRU.h
uint32_t audioOutChannels
Number of output audio channels.
Definition: PRU.h:61
uint32_t analogOutChannels
Number of output analog channels.
Definition: PRU.h:78
float digitalSampleRate
Digital sample rate in Hz (currently always 44100.0)
Definition: PRU.h:95
uint32_t digitalFrames
Number of digital frames per period.
Definition: PRU.h:89
uint32_t audioInChannels
Number of input audio channels.
Definition: PRU.h:59
uint32_t audioFrames
Number of audio frames per period.
Definition: PRU.h:57
uint32_t digitalChannels
Number of digital channels.
Definition: PRU.h:93
uint32_t * digital
Buffer holding digital input/output samples.
Definition: PRU.h:54
uint32_t analogFrames
Number of analog frames per period.
Definition: PRU.h:68
Definition: PRU.h:117
uint32_t analogInChannels
Number of input analog channels.
Definition: PRU.h:73
float * analogIn
Buffer holding analog input samples.
Definition: PRU.h:42
float * analogOut
Buffer holding analog output samples.
Definition: PRU.h:49
float * audioOut
Buffer holding audio output samples.
Definition: PRU.h:35
float audioSampleRate
Audio sample rate in Hz (currently always 44100.0)
Definition: PRU.h:63
uint64_t audioFramesElapsed
Number of elapsed audio frames since the start of rendering.
Definition: PRU.h:103
float analogSampleRate
Analog sample rate in Hz.
Definition: PRU.h:86
Definition: PRU.h:22
Generated on Mon Mar 10 2025 06:27:56 for Bela by
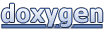