x
|
vibrato.h
Go to the documentation of this file.
90 void DeFM(double* f2, double* f1, double* AA, int npfr, int* peakfr, int Fr, double& fct, int& fcount, double* &frs, double* &f, bool furthersmoothing=false);
91 void DeFM(double* f2, double* f1, double* AA, int npfr, double* lp, int Fr, double& fct, int& fcount, double* &frs, double* &f, bool furthersmoothing=false);
96 double S_F(int afres, double* LogAF, double* LogAS, int Fr, int M, atom** Partials, double* A0C, double* lp, int P, double F0Overall);
97 double S_F_SV(int afres, double* LogAF, double* LogAS, int Fr, int M, atom** Partials, double* A0C, double* lp, int P, double F=0.005, int FScaleMode=0, double theta=0.5, double sps=44100);
100 void AnalyzeV(THS& HS, TVo& V, double*& peaky, double*& cyclefrs, double*& cyclefs, double sps, double h, int* cyclefrcount=0,
107 void FindPeaks(int* peakfr, int& npfr, double* F0, int Fr, double periodinframe, double*& peaky);
108 void FS_QR(int& K, double* FXR, double* data, int Fr, double period, double shift, double* FRES);
109 void RateAndReg(double& rate, double& regularity, double* data, int frst, int fren, int padrate, double sps, double offst);
void FindPeaks(int *peakfr, int &npfr, double *F0, int Fr, double periodinframe, double *&peaky)
Definition: vibrato.cpp:588
void DeFM(double *f2, double *f1, double *AA, int npfr, int *peakfr, int Fr, double &fct, int &fcount, double *&frs, double *&f, bool furthersmoothing=false)
Definition: vibrato.cpp:496
double S_F(int afres, double *LogAF, double *LogAS, int Fr, int M, atom **Partials, double *A0C, double *lp, int P, double F0Overall)
Definition: vibrato.cpp:1055
Definition: vibrato.h:35
Definition: hs.h:49
double * lp
[0:P-1] peak positions, refined to floating-point values in frames
Definition: vibrato.h:48
void RateAndReg(double &rate, double ®ularity, double *data, int frst, int fren, int padrate, double sps, double offst)
Definition: vibrato.cpp:918
void FS_QR(int &K, double *FXR, double *data, int Fr, double period, double shift, double *FRES)
Definition: vibrato.cpp:759
Definition: hs.h:147
double S_F_SV(int afres, double *LogAF, double *LogAS, int Fr, int M, atom **Partials, double *A0C, double *lp, int P, double F=0.005, int FScaleMode=0, double theta=0.5, double sps=44100)
Definition: vibrato.cpp:1182
double ** fxr
[1:P-1][0:2K] single-cycle modulator shape coefficients - cosine, sine, cosine, ...
Definition: vibrato.h:51
void AnalyzeV(THS &HS, TVo &V, double *&peaky, double *&cyclefrs, double *&cyclefs, double sps, double h, int *cyclefrcount=0, int SFMode=0, double SFF=0.01, int SFFScale=0, double SFtheta=0)
Definition: vibrato.cpp:196
Generated on Sat Apr 26 2025 07:05:12 for x by
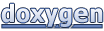