x
|
#include "xcomplex.h"
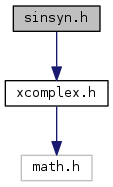
Go to the source code of this file.
Functions | |
void | CosSin (double *datar, double *datai, int CountSt, int CountEn, double f3, double f2, double f1, double f0, double &ph) |
void | Sinusoid (double *data, int T, double a1, double a2, double f1, double f2, double p1, double p2, double *a, double *f, double *p, bool ad=true) |
void | Sinusoid (double *data, int T, double a1, double a2, double f1, double f2, double p1, double p2, bool ad=true) |
void | Sinusoid (int T, double *f, double *a, double *ph, double *da, double aa, double ab, double ac, double ad, double fa, double fb, double fc, double fd, double ph0, double ph2, bool LogA=false) |
void | Sinusoid (int T, double *f, double *ph, double fa, double fb, double fc, double fd, double ph0, double ph2) |
void | Sinusoid (int T, double *data, double a3, double a2, double a1, double a0, double f3, double f2, double f1, double f0, double ph0, double ph2, bool add) |
void | Sinusoid (double *data, int CountSt, int CountEn, double a3, double a2, double a1, double a0, double f3, double f2, double f1, double f0, double &ph, bool add) |
void | Sinusoid_direct (double *f, double *a, double *ph, double *da, int CountSt, int CountEn, double aa, double ab, double ac, double ad, double fa, double fb, double fc, double fd, double &p1, bool LogA=false) |
void | Sinusoid_direct (double *f, double *ph, int CountSt, int CountEn, double fa, double fb, double fc, double fd, double &p1) |
void | SinusoidExp (cdouble *data, int CountSt, int CountEn, double a3, double a2, double a1, double a0, double omg3, double omg2, double omg1, double omg0, double &ea, double &ph, bool add) |
void | SinusoidExp (int T, cdouble *s, cdouble *ds, int M, cdouble *lamda, double **h, double **dih, cdouble &tmpexp) |
void | SinusoidExp (int T, cdouble *s, int M, cdouble *lamda, double **dih, cdouble &tmpexp) |
void | SinusoidExpA (cdouble *data, int CountSt, int CountEn, double a3, double a2, double a1, double a0, double omg3, double omg2, double omg1, double omg0, double &ph, bool add) |
void | SinusoidExpA (int T, cdouble *s, cdouble *ds, int M, double *p, double *q, double **h, double **dh, double **dih, double &tmpph) |
void | SinusoidExpA (int T, cdouble *s, int M, double *p, double *q, double **h, double **dih, double &tmpph) |
void | SinusoidExpA (int T, cdouble *s, int M, double *p, double *q, double **h, double **dih, double ph1, double ph2) |
void | ShiftTrinomial (double T, double &b3, double &b2, double &b1, double &b0, double a3, double a2, double a1, double a0) |
double * | SynthesizeSinusoid (double *xrec, int dst, int den, double *phs, int Fr, double *xs, double *fs, double *as, bool add, bool *terminatetag) |
double * | SynthesizeSinusoidP (double *xrecm, int dst, int den, double *phs, int Fr, double *xs, double *fs, double *as, bool add) |
Detailed Description
- sinusoid synthesis routines for sinusoid modeling cycle. Functions named Sinusoid construct sinusoid segments whose amplitude and frequency are modeled as polynomials or linear combinations of basis functions; functions named SinusoidExp construct sinusoid segments whose logarithmic amplitude derivative and frequency are modeled thus; functions named SinusoidExpA construct sinusoid segments whose log amplitude and frequency are modeled thus.
Function Documentation
void CosSin | ( | double * | datar, |
double * | datai, | ||
int | CountSt, | ||
int | CountEn, | ||
double | f3, | ||
double | f2, | ||
double | f1, | ||
double | f0, | ||
double & | ph | ||
) |
function CosSin: recursive cos-sin generator with trinomial frequency
In: CountSt, CountEn f3, f2, f1, f0: trinomial coefficients of frequency ph: initial phase angle at 0 (NOT at CountSt) Out: datar[CountSt:CountEn-1], datai[CountSt:CountEn-1]: synthesized pair of cosine and sine functions ph: phase angle at CountEn
No return value.
void ShiftTrinomial | ( | double | T, |
double & | b3, | ||
double & | b2, | ||
double & | b1, | ||
double & | b0, | ||
double | a3, | ||
double | a2, | ||
double | a1, | ||
double | a0 | ||
) |
function ShiftTrinomial: shifts the origin of a trinomial from 0 to T
In: a3, a2, a1, a0. Out: b3, b2, b1, b0, so that a3*x^3+a2*x^2+a1*x+a0=b3(x-T)^3+b2(x-T)^2+b1(x-T)+b0
No return value.
void Sinusoid | ( | double * | data, |
int | T, | ||
double | a1, | ||
double | a2, | ||
double | f1, | ||
double | f2, | ||
double | p1, | ||
double | p2, | ||
double * | a, | ||
double * | f, | ||
double * | p, | ||
bool | ad | ||
) |
function Sinuoid: original McAuley-Quatieri synthesizer interpolation between two measurement points.
In: T: length from measurement point 1 to measurement point 2 a1, f1, p2: amplitude, frequency and phase angle at measurement point 1 a2, f2, p2: amplitude, frequency and phase angle at measurement point 2 ad: specifies if the resynthesized sinusoid is to be added to or to replace the contents of output buffer Out: data[T]: output buffer a[T], f[T], p[T]: resynthesized amplitude, frequency and phase
No return value.
void Sinusoid | ( | double * | data, |
int | T, | ||
double | a1, | ||
double | a2, | ||
double | f1, | ||
double | f2, | ||
double | p1, | ||
double | p2, | ||
bool | ad | ||
) |
function Sinuoid: original McAuley-Quatieri synthesizer interpolation between two measurement points, without returning interpolated sinusoid parameters.
In: T: length from measurement point 1 to measurement point 2 a1, f1, p2: amplitude, frequency and phase angle at measurement point 1 a2, f2, p2: amplitude, frequency and phase angle at measurement point 2 ad: specifies if the resynthesized sinusoid is to be added to or to replace the contents of output buffer Out: data[T]: output buffer
No return value.
void Sinusoid | ( | int | T, |
double * | f, | ||
double * | a, | ||
double * | ph, | ||
double * | da, | ||
double | aa, | ||
double | ab, | ||
double | ac, | ||
double | ad, | ||
double | fa, | ||
double | fb, | ||
double | fc, | ||
double | fd, | ||
double | ph0, | ||
double | ph2, | ||
bool | LogA | ||
) |
function Sinusoid: synthesizes sinusoid piece from trinomial frequency and amplitude coefficients, returning sinusoid coefficients instead of waveform.
In: T aa, ab, ac, ad: trinomial coefficients of amplitude (or log amplitude if LogA=true) fa, fb, fc, fd: trinomial coefficients of frequency. ph0, ph2: phase angles at 0 and CountEn. LogA: specifies whether log amplitude or amplitude is a trinomial Out: f[CountSt:CountEn-1], a[CountSt:CountEn-1], ph[CountSt:CountEn-1]: synthesized sinusoid parameters da[CountSt:CountEn-1]: derivative of synthesized amplitude, optional
No return value.
void Sinusoid | ( | int | T, |
double * | f, | ||
double * | ph, | ||
double | fa, | ||
double | fb, | ||
double | fc, | ||
double | fd, | ||
double | ph0, | ||
double | ph2 | ||
) |
function Sinusoid: generates trinomial frequency and phase with phase correction.
In: T fa, fb, fc, fd: trinomial coefficients of frequency. ph0, ph2: phase angles at 0 and CountEn. Out: f[T], ph[T]: output buffers holding frequency and phase.
No return value.
void Sinusoid | ( | int | T, |
double * | data, | ||
double | aa, | ||
double | ab, | ||
double | ac, | ||
double | ad, | ||
double | fa, | ||
double | fb, | ||
double | fc, | ||
double | fd, | ||
double | ph0, | ||
double | ph2, | ||
bool | add | ||
) |
function Sinusoid: synthesizes sinusoid piece from trinomial frequency and amplitude coefficients.
In: T aa, ab, ac, ad: trinomial coefficients of amplitude. fa, fb, fc, fd: trinomial coefficients of frequency. ph0, ph2: phase angles at 0 and CountEn. add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: data[T]: output buffer.
No return value.
void Sinusoid | ( | double * | data, |
int | CountSt, | ||
int | CountEn, | ||
double | a3, | ||
double | a2, | ||
double | a1, | ||
double | a0, | ||
double | f3, | ||
double | f2, | ||
double | f1, | ||
double | f0, | ||
double & | ph, | ||
bool | add | ||
) |
function Sinusoid: synthesizes sinusoid over [CountSt, CountEn) from tronomial coefficients of amplitude and frequency, recursive implementation.
In: CountSt, CountEn a3, a2, a1, a0: trinomial coefficients of amplitude f3, f2, f1, f0: trinomial coefficients of frequency ph: initial phase angle at 0 (NOT at CountSt) add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: data[CountSt:CountEn-1]: output buffer. ph: phase angle at CountEn
No return value. This function requires 8-byte stack alignment for optimal speed.
void Sinusoid_direct | ( | double * | f, |
double * | a, | ||
double * | ph, | ||
double * | da, | ||
int | CountSt, | ||
int | CountEn, | ||
double | aa, | ||
double | ab, | ||
double | ac, | ||
double | ad, | ||
double | fa, | ||
double | fb, | ||
double | fc, | ||
double | fd, | ||
double & | p1, | ||
bool | LogA | ||
) |
function Sinusoid_direct: synthesizes sinusoid over [CountSt, CountEn) from tronomial coefficients of amplitude and frequency, direct implementation returning sinusoid coefficients rather than waveform.
In: CountSt, CountEn aa, ab, ac, ad: trinomial coefficients of amplitude fa, fb, fc, fd: trinomial coefficients of frequency p1: initial phase angle at 0 (NOT at CountSt) Out: f[CountSt:CountEn-1], a[:], ph[:], da[:]: output buffers. p1: phase angle at CountEn
No return value.
void Sinusoid_direct | ( | double * | f, |
double * | ph, | ||
int | CountSt, | ||
int | CountEn, | ||
double | fa, | ||
double | fb, | ||
double | fc, | ||
double | fd, | ||
double & | p1 | ||
) |
function Sinusoid_direct: synthesizes sinusoid over [CountSt, CountEn) from tronomial coefficients of frequency, direct implementation returning frequency and phase rather than waveform.
In: CountSt, CountEn fa, fb, fc, fd: trinomial coefficients of frequency p1: initial phase angle at 0 (NOT at CountSt) Out: f[CountSt:CountEn-1], ph[CountSt:CountEn-1]: output buffers. p1: phase angle at CountEn
No return value.
void SinusoidExp | ( | cdouble * | data, |
int | CountSt, | ||
int | CountEn, | ||
double | a3, | ||
double | a2, | ||
double | a1, | ||
double | a0, | ||
double | omg3, | ||
double | omg2, | ||
double | omg1, | ||
double | omg0, | ||
double & | ea, | ||
double & | ph, | ||
bool | add | ||
) |
function SinusoidExp: synthesizes complex sinusoid whose derivative log amplitude and frequency are trinomials
In: CountSt, CountEn a3, a2, a1, a0: trinomial coefficients for the derivative of log amplitude omg3, omg2, omg1, omg0: trinomial coefficients for angular frequency ea, ph: initial log amplitude and phase angle at 0 add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: data[CountSt:CountEn-1]: output buffer. ea, ph: log amplitude and phase angle at CountEn.
No return value.
void SinusoidExp | ( | int | T, |
cdouble * | s, | ||
cdouble * | ds, | ||
int | M, | ||
cdouble * | lamda, | ||
double ** | h, | ||
double ** | dih, | ||
cdouble & | tmpexp | ||
) |
function SinusoidExp: synthesizes complex sinusoid piece whose derivative logarithm is h[M]'lamda[M]. This version also synthesizes its derivative.
In: h[M][T], dih[M][T]: basis functions and their difference-integrals lamda[M]: coefficients of h[M] tmpexp: inital logarithm at 0 Out: s[T], ds[T]: synthesized sinusoid and its derivative tmpexp: logarithm at T
No return value.
function SinusoidExp: synthesizes complex sinusoid piece whose derivative logarithm is h[M]'lamda[M]. This version does not synthesize its derivative.
In: dih[M][T]: difference of integrals of basis functions h[M] lamda[M]: coefficients of h[M] tmpexp: inital logarithm at 0 Out: s[T]: synthesized sinusoid tmpexp: logarithm at T
No return value.
void SinusoidExpA | ( | cdouble * | data, |
int | CountSt, | ||
int | CountEn, | ||
double | a3, | ||
double | a2, | ||
double | a1, | ||
double | a0, | ||
double | omg3, | ||
double | omg2, | ||
double | omg1, | ||
double | omg0, | ||
double & | ph, | ||
bool | add | ||
) |
function SinusoidExpA: synthesizes complex sinusoid whose log amplitude and frequency are trinomials
In: CountSt, CountEn a3, a2, a1, a0: trinomial coefficients for log amplitude omg3, omg2, omg1, omg0: trinomial coefficients for angular frequency ph: initial phase angle at 0 add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: data[CountSt:CountEn-1]: output buffer. ph: phase angle at CountEn.
No return value.
void SinusoidExpA | ( | int | T, |
cdouble * | s, | ||
cdouble * | ds, | ||
int | M, | ||
double * | p, | ||
double * | q, | ||
double ** | h, | ||
double ** | dh, | ||
double ** | dih, | ||
double & | tmpph | ||
) |
function SinusoidExpA: synthesizes complex sinusoid piece whose log amplitude is h[M]'p[M] and frequency is h[M]'q[M]. This version also synthesizes its derivative.
In: h[M][T], dh[M][T], dih[M][T]: basis functions and their derivatives and difference-integrals p[M], q[M]: real and imaginary parts of coefficients of h[M] tmpph: inital phase angle at 0 Out: s[T], ds[T]: synthesized sinusoid and its derivative tmpph: phase angle at T
No return value.
void SinusoidExpA | ( | int | T, |
cdouble * | s, | ||
int | M, | ||
double * | p, | ||
double * | q, | ||
double ** | h, | ||
double ** | dih, | ||
double & | tmpph | ||
) |
function SinusoidExpA: synthesizes complex sinusoid piece whose log amplitude is h[M]'p[M] and frequency is h[M]'q[M]. This version does not synthesize its derivative.
In: h[M][T], dih[M][T]: basis functions and their difference-integrals p[M], q[M]: real and imaginary parts of coefficients of h[M] tmpph: inital phase angle at 0 Out: s[T]: synthesized sinusoid tmpph: phase angle at T
No return value.
void SinusoidExpA | ( | int | T, |
cdouble * | s, | ||
int | M, | ||
double * | p, | ||
double * | q, | ||
double ** | h, | ||
double ** | dih, | ||
double | ph1, | ||
double | ph2 | ||
) |
function SinusoidExpA: synthesizes complex sinusoid piece whose log amplitude is h[M]'p[M] and frequency is h[M]'q[M] with phase angle specified at both ends. This version does not synthesize its derivative.
In: h[M][T], dih[M][T]: basis functions and their difference-integrals p[M], q[M]: real and imaginary parts of coefficients of h[M] ph1, ph2: phase angles at 0 and T. Out: s[T]: synthesized sinusoid
No return value.
double* SynthesizeSinusoid | ( | double * | xrec, |
int | dst, | ||
int | den, | ||
double * | phs, | ||
int | Fr, | ||
double * | xs, | ||
double * | fs, | ||
double * | as, | ||
bool | add, | ||
bool * | terminatetag | ||
) |
function SynthesizeSinusoid: synthesizes a time-varying sinusoid from a sequence of frequencies and amplitudes
In: xs[Fr]: measurement points, should be integers although *xs has double type. fs[Fr], as[Fr]: sequence of frequencies and amplitudes at xs[Fr] phs[0]: initial phase angle at (int)xs[0]. dst, den: start and end time of synthesis, dst<=xs[0], den>=xs[Fr-1] add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: xrec[0:den-dst-1]: output buffer hosting synthesized sinusoid from dst to den. phs[Fr]: phase angles at xs[Fr]
Returns pointer to xrec.
double* SynthesizeSinusoidP | ( | double * | xrecm, |
int | dst, | ||
int | den, | ||
double * | phs, | ||
int | Fr, | ||
double * | xs, | ||
double * | fs, | ||
double * | as, | ||
bool | add | ||
) |
function SynthesizeSinusoidP: synthesizes a time-varying sinusoid from a sequence of frequencies, amplitudes and phase angles
In: xs[Fr]: measurement points, should be integers although *xs has double type. fs[Fr], as[Fr], phs[Fr]: sequence of frequencies, amplitudes and phase angles at xs[Fr] dst, den: start and end time of synthesis, dst<=xs[0], den>=xs[Fr-1] add: specifies if the resynthesized sinusoid is to be added to or to replace the content of output buffer Out: xrecm[0:den-dst-1]: output buffer hosting synthesized sinusoid from dst to den.
Returns pointer to xrecm.
Generated on Sat Apr 26 2025 07:05:12 for x by
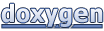