x
|
Go to the source code of this file.
Functions | |
double | nextdouble (double x, double dir) |
double | GradientMax (void *params, double(*F)(int, double *, void *), void(*dF)(double *, int, double *, void *), int dim, double *start, double ep=1e-6, int maxiter=100) |
double | GradientOneMax (void *params, double(*F)(int, double *, void *), void(*dF)(double *, int, double *, void *), int dim, double *start, double ep, int maxiter) |
double | Newton (double &x0, double(*y)(double, void *), double(*y1)(double, void *), void *params=0, double epx=1e-6, int maxiter=100, double epy=1e-256) |
int | Newton (double &x0, double(*dy)(double, void *), void *params, int yshift, double epx=1e-6, int maxiter=100, double epy=1e-256, double xmin=-1e308, double xmax=1e308) |
double | Newton1dmax (double &x0, double xa, double xb, double(*ddy)(double, void *), void *params, int y1shift, int yshift, double(*dy)(double, void *), int dyshift, double epx=1e-6, int maxiter=100, double epdy=1e-128, bool checkinput=true) |
double | Newton2D (double &x1, double &x2, double(*dy)(double &, double &, void *), double(*ddy)(double &, double &, double &, void *), void *params=0, double epx1=1e-6, double epx2=1e-6, int maxiter=100, double epdy=1e-256) |
double | rootsearchhalf (double &x1, double x2, double(*y)(double, void *), void *params=0, double epx=1e-6, int maxiter=100) |
double | rootsearchsecant (double &x1, double x2, double(*y)(double, void *), void *params=0, double epx=1e-6, int maxiter=100) |
double | rootsearchbisecant (double &x1, double x2, double(*y)(double, void *), void *params=0, double epx=1e-6, int maxiter=100) |
double | Search1Dmax (double &x, void *params, double(*F)(double, void *), double inf, double sup, double *maxresult=0, double ep=1e-6, int maxiter=100, bool convcheck=false) |
double | Search1DmaxEx (double &x, void *params, double(*F)(double, void *), double inf, double sup, double *maxresult=0, double ep=1e-6, int maxiter=100, double len=1) |
double | Search1Dmaxfrom (void *params, double(*F)(int, double *, void *), int dim, double *start, double *direct, double step=1e-2, double minstep=1e-6, double maxstep=1e6, double *opt=0, double *max=0, double ep=1e-6, int maxiter=100, bool convcheck=false) |
Detailed Description
- optimization routines
Function Documentation
double GradientMax | ( | void * | params, |
double(*)(int, double *, void *) | F, | ||
void(*)(double *, int, double *, void *) | dF, | ||
int | dim, | ||
double * | start, | ||
double | ep, | ||
int | maxiter | ||
) |
function GradientMax: gradient method maximizing F(x)
In: function F(x) and its derivative dF(x) start[dim]: initial value of x ep: a stopping threshold maxiter: maximal number of iterates Out: start[dim]: the final x
Returnx max F(final x)
double GradientOneMax | ( | void * | params, |
double(*)(int, double *, void *) | F, | ||
void(*)(double *, int, double *, void *) | dF, | ||
int | dim, | ||
double * | start, | ||
double | ep, | ||
int | maxiter | ||
) |
function GradientOneMax: gradient method maximizing F(x), which searches $dim dimensions one after another instead of taking the gradient descent direction.
In: function F(x) and its derivative dF(x) start[dim]: initial value of x ep: a stopping threshold maxiter: maximal number of iterates Out: start[dim]: the final x
Returnx max F(final x)
double Newton | ( | double & | x0, |
double(*)(double, void *) | y, | ||
double(*)(double, void *) | y1, | ||
void * | params, | ||
double | epx, | ||
int | maxiter, | ||
double | epy | ||
) |
function Newton: Newton method for finding the root of y(x)
In: function y(x) and its derivative y'(x) params: additional argument for calling y and y' x0: inital value of x epx, epy: stopping thresholds on x and y respectively maxiter: maximal number of iterates Out: x0: the root
Returns 0 if successful.
int Newton | ( | double & | x0, |
double(*)(double, void *) | dy, | ||
void * | params, | ||
int | yshift, | ||
double | epx, | ||
int | maxiter, | ||
double | epy, | ||
double | xmin, | ||
double | xmax | ||
) |
function Newton: Newton method for finding the root of y(x), for use when it's more efficient for y' and y to be calculated in one function call
In: function dy(x) that computes y and y' at x params: additional argument for calling dy yshift: the byte shift in params where dy(x) put the value of y(x) as double type x0: inital value of x epx, epy: stopping thresholds on x and y respectively maxiter: maximal number of iterates Out: x0: the root
Returns 0 if successful.
double Newton1dmax | ( | double & | x0, |
double | xa, | ||
double | xb, | ||
double(*)(double, void *) | ddy, | ||
void * | params, | ||
int | y1shift, | ||
int | yshift, | ||
double(*)(double, void *) | dy, | ||
int | dyshift, | ||
double | epx, | ||
int | maxiter, | ||
double | epdy, | ||
bool | checkinput | ||
) |
function Newton1dmax: uses init_Newton_1d_max and Newton_1d_max to search for a local maximum of y.
By default ddy and dy use the same parameter structure and returns the lower order derivatives at specific offsets within that structure. However, since the same params is used, it's possible the same variable may take different offsets when returned from ddy() and dy().
On calling xa<x0<xb, and it is assumed that y0>=ya, y0>=yb. If the conditions on y are not met, it returns a negative value showing the order of y0, ya and yb, i.e. -0.5 for ya<=y0<yb -0.6 for yb<=y0<ya -0.7 for y0<ya<yb -0.8 for y0<yb<=ya
In: function ddy(x) that computes y, y' and y" at x function dy(x) that computes y and y' at x params: additional argument for calling ddy and dy y1shift: the byte shift in params where ddy(x) put the value of y'(x) as double type yshift: the byte shift in params where ddy(x) put the value of y(x) as double type dyshift: the byte shift in params where dy(x) put the value of y(x) as double type x0: inital value of x (xa, xb): initial search range epx, epdy: stopping threshold for x and y', respectively maxiter: maximal number of iterates Out: x0: the maximum
Returns the error bound for maximum x0, or a negative value if the initial point does not satisfy relevent assumptions.
double rootsearchbisecant | ( | double & | x1, |
double | x2, | ||
double(*)(double, void *) | y, | ||
void * | params, | ||
double | epx, | ||
int | maxiter | ||
) |
function rootsearchbisecant: searches for the root of y(x) in (x1, x2) by bi-secant method. On calling given that y(x1) and y(x2) have different signs. The bound interval (x1, x2) converges to 0, as compared to standared secant method.
In: function y(x), params: additional arguments for calling y (x1, x2): inital range epx: stopping threshold on x maxiter: maximal number of iterates Out: root x1
Returns an estimated error bound if a root is found, a negative value if not.
double rootsearchhalf | ( | double & | x1, |
double | x2, | ||
double(*)(double, void *) | y, | ||
void * | params, | ||
double | epx, | ||
int | maxiter | ||
) |
function rootsearchhalf: searches for the root of y(x) in (x1, x2) by half-split method. On calling y(x1) and y(x2) must not have the same sign, or -1 is returned signaling a failure.
In: function y(x), params: additional arguments for calling y (x1, x2): inital range epx: stopping threshold on x maxiter: maximal number of iterates Out: root x1
Returns a positive error estimate if a root is found, -1 if not.
double rootsearchsecant | ( | double & | x1, |
double | x2, | ||
double(*)(double, void *) | y, | ||
void * | params, | ||
double | epx, | ||
int | maxiter | ||
) |
function rootsearchsecant: searches for the root of y(x) in (x1, x2) by secant method.
In: function y(x), params: additional arguments for calling y (x1, x2): inital range epx: stopping threshold on x maxiter: maximal number of iterates Out: root x1
Returns a positive error estimate if a root is found, -1 if not.
double Search1Dmax | ( | double & | x, |
void * | params, | ||
double(*)(double, void *) | F, | ||
double | inf, | ||
double | sup, | ||
double * | maxresult, | ||
double | ep, | ||
int | maxiter, | ||
bool | convcheck | ||
) |
function Search1Dmax: 1-dimensional maximum search within interval [inf, sup] using 0.618 method
In: function F(x), params: additional arguments for calling F (inf, sup): inital range epx: stopping threshold on x maxiter: maximal number of iterates convcheck: specifies if convexity is checked at each interate, false recommended. Out: x: the maximum maxresult: the maximal value, if specified
Returns an estimated error bound of x if successful, a negative value if not.
double Search1DmaxEx | ( | double & | x, |
void * | params, | ||
double(*)(double, void *) | F, | ||
double | inf, | ||
double | sup, | ||
double * | maxresult, | ||
double | ep, | ||
int | maxiter, | ||
double | len | ||
) |
function Search1DmaxEx: 1-dimensional maximum search using Search1Dmax, but allows setting a $len argument so that a pre-location of the maximum is performed by sampling (inf, sup) at interval $len, so that Search1Dmax will work on an interval no longer than 2*len.
In: function F(x), params: additional arguments for calling F (inf, sup): inital range len: rough sampling interval for pre-locating maximum epx: stopping threshold on x maxiter: maximal number of iterates convcheck: specifies if convexity is checked at each interate, false recommended. Out: x: the maximum maxresult: the maximal value, if specified
Returns an estimated error bound of x if successful, a negative value if not.
double Search1Dmaxfrom | ( | void * | params, |
double(*)(int, double *, void *) | F, | ||
int | dim, | ||
double * | start, | ||
double * | direct, | ||
double | step, | ||
double | minstep, | ||
double | maxstep, | ||
double * | opt, | ||
double * | max, | ||
double | ep, | ||
int | maxiter, | ||
bool | convcheck | ||
) |
function Search1Dmaxfrom: 1-dimensional maximum search from start[] towards start+direct[]. The function tries to locate an interval ("step") for which the 4-point convexity rule confirms the existence of a convex maximum.
In: function F(x) params: additional arguments for calling F start[dim]: initial x[] direct[dim]: search direction step: initial step size minstep: minimal step size in 4-point scheme between x0 and x3 maxstep: maximal step size in 4-point scheme between x0 and x3 Out: opt[dim]: the maximum, if specified max: maximal value, if specified
Returns x>0 that locally maximizes F(start+x*direct) or x|-x>0 that maximizes F(start-x*direct) at calculated points if convex check fails.
Generated on Wed Jun 18 2025 07:06:21 for x by
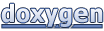