svapp
1.9
|
VersionTester.h
Go to the documentation of this file.
static bool isVersionNewerThan(QString, QString)
Definition: VersionTester.cpp:54
Definition: VersionTester.h:31
void newerVersionAvailable(QString)
VersionTester(QString hostname, QString versionFilePath, QString myVersion)
Definition: VersionTester.cpp:29
Generated by
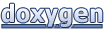