qm-dsp
1.8
|
cluster_segmenter.h
Go to the documentation of this file.
void cluster_segment(int *q, double **features, int frames_read, int feature_length, int nHMM_states, int histogram_length, int nclusters, int neighbour_limit)
void constq_segment(int *q, double **features, int frames_read, int bins, int ncoeff, int feature_type, int nHMM_states, int histogram_length, int nclusters, int neighbour_limit)
void cq2chroma(double **cq, int nframes, int ncoeff, int bins, double **chroma)
void mpeg7_constq(double **features, int nframes, int ncoeff)
void create_histograms(int *x, int nx, int m, int hlen, double *h)
Generated by
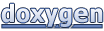