FFmpeg
|
stpt2.m
Go to the documentation of this file.
38 ploc=1+find((mX(2:N2-1)>t).*(mX(2:N2-1)>mX(3:N2)).*(mX(2:N2-1)>mX(1:N2-2))); %Peak finder above threshold (t)
N, 1 zeros()
FFT size for synthesis(even) H
Definition: libstagefright.cpp:45
MUSIC TECHNOLOGY GROUP UNIVERSITAT POMPEU FABRA Free Non Commercial Binary License Agreement UNIVERSITAT POMPEU OR INDICATING ACCEPTANCE BY SELECTING THE ACCEPT BUTTON ON ANY DOWNLOAD OR INSTALL YOU ACCEPT THE TERMS OF THE LICENSE SUMMARY TABLE Software MELODIA Melody Extraction vamp plug in Licensor Music Technology Group Universitat Pompeu Plaça de la Spain Permitted purposes Non commercial internal research and validation and educational purposes only All commercial uses in a production either internal or are prohibited by this license and require an additional commercial exploitation license TERMS AND CONDITIONS SOFTWARE Software means the software programs identified herein in binary any other machine readable any updates or error corrections provided by and any user programming guides and other documentation provided to you by UPF under this Agreement LICENSE Subject to the terms and conditions of this UPF grants you a royalty non non non limited for the specified duration in to reproduce and use internally Software complete and unmodified for the purposes indicated in the Summary Table CONDITIONS The Software is confidential and copyrighted You may make one backup copy Unless enforcement is prohibited by applicable law and other than as expressly provided in the Table above
Definition: MELODIA - License.txt:16
sound(x3, Fs)
1i.*Xphase exp()
fftbuffer, N fft()
1:W2 xw()
static uint32_t inverse(uint32_t v)
find multiplicative inverse modulo 2 ^ 32
Definition: asfcrypt.c:35
Generated on Fri May 30 2025 06:54:02 for FFmpeg by
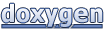