GPU Multirate FIR Filter
|
filters.h
Go to the documentation of this file.
78 void compute_ref( float *h_in[], float *h_reference[], gpu_arrays *gpuarrays, params *gparams, cmd_args *args, filter_arrays *farr, int N);
80 void compute_omp(float *h_in[], float *h_reference[], gpu_arrays *gpuarrays, params *gparams, cmd_args *args, filter_arrays *farr, int N);
82 void check_results(float *h_reference[], float *h_out[], gpu_arrays *gpuarrays, params *gparams, int N);
94 void cudaMultiFilterFirInit(float **in, float **out, float *filters, params *params, gpu_arrays *arrays);
void read_command_line(int argc, char *argv[], cmd_args *args)
Definition: filters_host.cpp:231
Definition: filters.h:67
void compute_omp(float *h_in[], float *h_reference[], gpu_arrays *gpuarrays, params *gparams, cmd_args *args, filter_arrays *farr, int N)
Definition: filters_host.cpp:123
Definition: filters.h:55
void compute_ref(float *h_in[], float *h_reference[], gpu_arrays *gpuarrays, params *gparams, cmd_args *args, filter_arrays *farr, int N)
Definition: filters_host.cpp:69
Definition: filters.h:48
void check_results(float *h_reference[], float *h_out[], gpu_arrays *gpuarrays, params *gparams, int N)
Definition: filters_host.cpp:188
Definition: filters.h:38
Generated on Thu Apr 24 2025 06:37:40 for GPU Multirate FIR Filter by
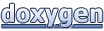