qm-dsp
1.8
|
DecimatorB carries out a fast downsample by a power-of-two factor. More...
#include <DecimatorB.h>
Public Member Functions | |
void | process (const double *src, double *dst) |
void | process (const float *src, float *dst) |
DecimatorB (int inLength, int decFactor) | |
Construct a DecimatorB to operate on input blocks of length inLength, with decimation factor decFactor. More... | |
virtual | ~DecimatorB () |
int | getFactor () const |
Private Member Functions | |
void | deInitialise () |
void | initialise (int inLength, int decFactor) |
void | doAntiAlias (const double *src, double *dst, int length, int filteridx) |
void | doProcess () |
Private Attributes | |
int | m_inputLength |
int | m_outputLength |
int | m_decFactor |
std::vector< std::vector< double > > | m_o |
double | m_a [7] |
double | m_b [7] |
double * | m_aaBuffer |
double * | m_tmpBuffer |
Detailed Description
DecimatorB carries out a fast downsample by a power-of-two factor.
It only knows how to decimate by a factor of 2, and will use repeated decimation for higher factors. A Butterworth filter of order 6 is used for the lowpass filter.
Definition at line 25 of file DecimatorB.h.
Constructor & Destructor Documentation
DecimatorB::DecimatorB | ( | int | inLength, |
int | decFactor | ||
) |
Construct a DecimatorB to operate on input blocks of length inLength, with decimation factor decFactor.
inLength should be a multiple of decFactor. Output blocks will be of length inLength / decFactor.
decFactor must be a power of two.
Definition at line 23 of file DecimatorB.cpp.
References initialise(), m_aaBuffer, m_decFactor, m_inputLength, m_outputLength, and m_tmpBuffer.
|
virtual |
Definition at line 34 of file DecimatorB.cpp.
References deInitialise().
Member Function Documentation
void DecimatorB::process | ( | const double * | src, |
double * | dst | ||
) |
Definition at line 131 of file DecimatorB.cpp.
References doProcess(), m_decFactor, m_inputLength, m_outputLength, and m_tmpBuffer.
void DecimatorB::process | ( | const float * | src, |
float * | dst | ||
) |
Definition at line 146 of file DecimatorB.cpp.
References doProcess(), m_decFactor, m_inputLength, m_outputLength, and m_tmpBuffer.
|
inline |
Definition at line 42 of file DecimatorB.h.
References deInitialise(), doAntiAlias(), doProcess(), initialise(), and m_decFactor.
|
private |
Definition at line 84 of file DecimatorB.cpp.
References m_aaBuffer, and m_tmpBuffer.
Referenced by getFactor(), and ~DecimatorB().
|
private |
Definition at line 39 of file DecimatorB.cpp.
References MathUtilities::isPowerOfTwo(), m_a, m_aaBuffer, m_b, m_decFactor, m_inputLength, m_o, m_outputLength, and m_tmpBuffer.
Referenced by DecimatorB(), and getFactor().
|
private |
Definition at line 90 of file DecimatorB.cpp.
Referenced by doProcess(), and getFactor().
|
private |
Definition at line 111 of file DecimatorB.cpp.
References doAntiAlias(), m_aaBuffer, m_decFactor, m_inputLength, and m_tmpBuffer.
Referenced by getFactor(), and process().
Member Data Documentation
|
private |
Definition at line 50 of file DecimatorB.h.
Referenced by DecimatorB(), doProcess(), initialise(), and process().
|
private |
Definition at line 51 of file DecimatorB.h.
Referenced by DecimatorB(), initialise(), and process().
|
private |
Definition at line 52 of file DecimatorB.h.
Referenced by DecimatorB(), doProcess(), getFactor(), initialise(), and process().
|
private |
Definition at line 54 of file DecimatorB.h.
Referenced by doAntiAlias(), and initialise().
|
private |
Definition at line 56 of file DecimatorB.h.
Referenced by doAntiAlias(), and initialise().
|
private |
Definition at line 57 of file DecimatorB.h.
Referenced by doAntiAlias(), and initialise().
|
private |
Definition at line 59 of file DecimatorB.h.
Referenced by DecimatorB(), deInitialise(), doProcess(), and initialise().
|
private |
Definition at line 60 of file DecimatorB.h.
Referenced by DecimatorB(), deInitialise(), doProcess(), initialise(), and process().
The documentation for this class was generated from the following files:
Generated by
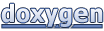