qm-dsp
1.8
|
DecimatorB.h
Go to the documentation of this file.
DecimatorB carries out a fast downsample by a power-of-two factor.
Definition: DecimatorB.h:25
DecimatorB(int inLength, int decFactor)
Construct a DecimatorB to operate on input blocks of length inLength, with decimation factor decFacto...
Definition: DecimatorB.cpp:23
void doAntiAlias(const double *src, double *dst, int length, int filteridx)
Definition: DecimatorB.cpp:90
Generated by
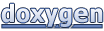